Possible Leak in OpenCV Threaded VideoCapture
在OpenCV中频繁开启销毁新线程并读取视频可能存在内存泄露的问题。
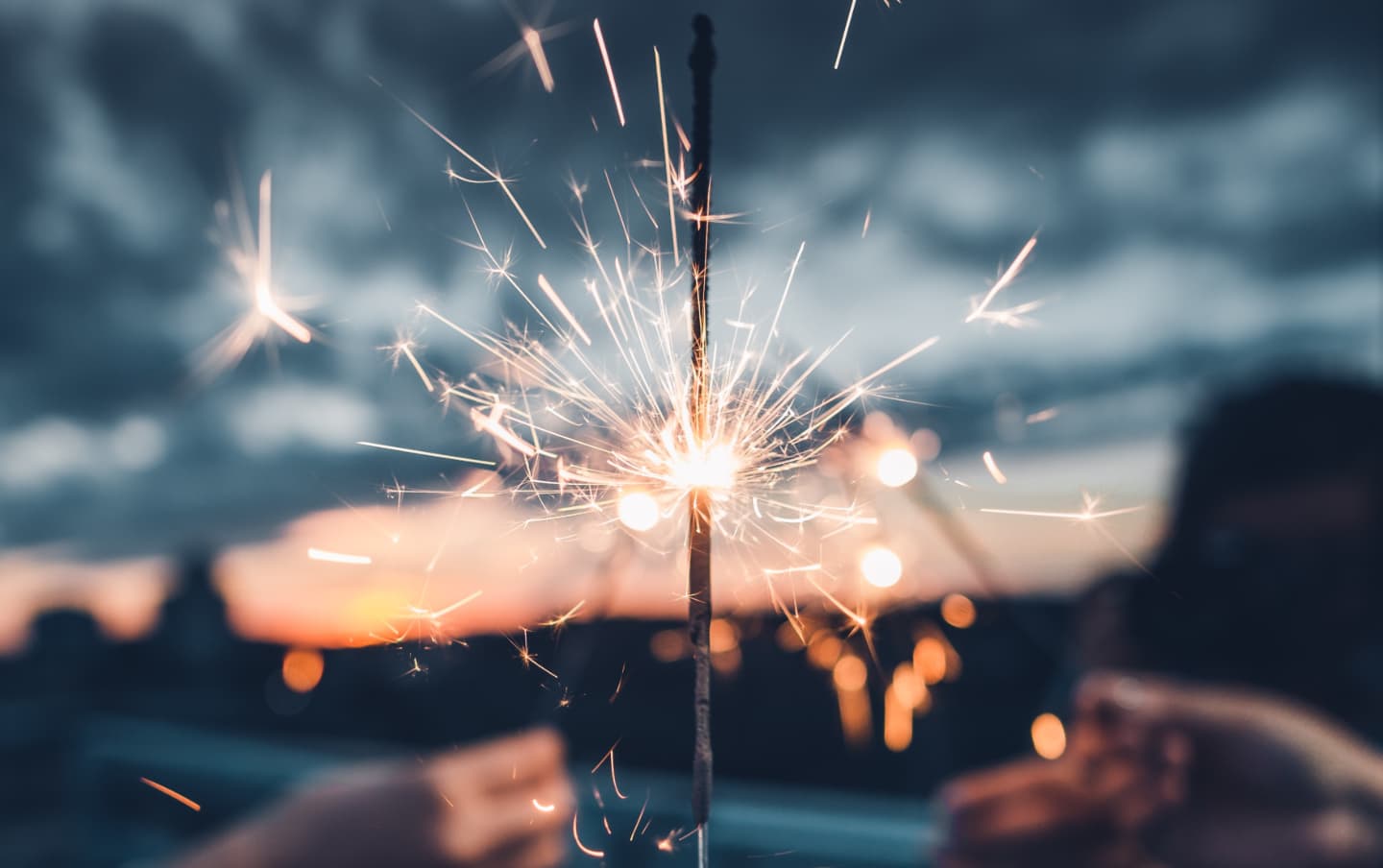
在OpenCV中频繁开启销毁新线程并读取视频可能存在内存泄露的问题。最小代码如下。
#include <chrono>
#include <fstream>
#include <iostream>
#include <opencv2/highgui.hpp>
#include <string.h>
#include <thread>
using namespace std;
void open_video(string const& video_url)
{
cv::VideoCapture cap(video_url);
cv::Mat frame;
cap.read(frame);
cap.release();
cout << "Video opened!" << endl;
}
int main(int argc, char** argv)
{
// Get args form input.
if (argc < 3) {
cout << "Usage: " << argv[0]
<< " video_file loop_times" << endl;
return -1;
}
string video_file = argv[1];
int loop_times = atoi(argv[2]);
// Loop through all the video files.
for (int i = 0; i < loop_times; i++) {
cout << "LOOP " << i << endl;
thread parse_video(open_video, ref(video_file));
parse_video.detach();
// sleep for a while, waitting for the thread.
this_thread::sleep_for(chrono::milliseconds(100));
}
return 0;
}
使用memory_profile记录内存的使用情况如下图所示。
2019-03-20更新:官方已经确认了leak的存在,issue地址:https://github.com/opencv/opencv/issues/13255
Comments ()