Rust使用进度条
命令行应用常用功能
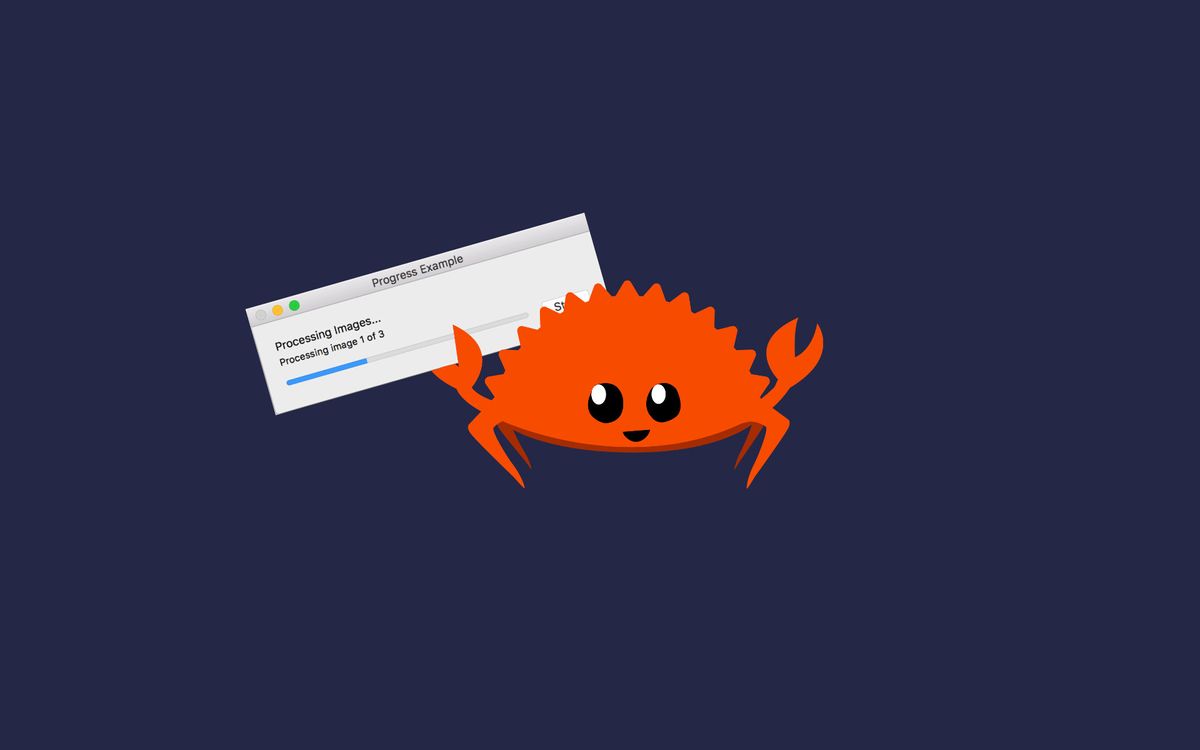
对于一些耗时操作,使用进度条可以提示用户程序正在运行中,显著改善用户体验。indicatif是Rust下的一个进度条crate,可以提供百分比、spinner等不同种类的进度条,还支持定义风格。先放一个官方示例:
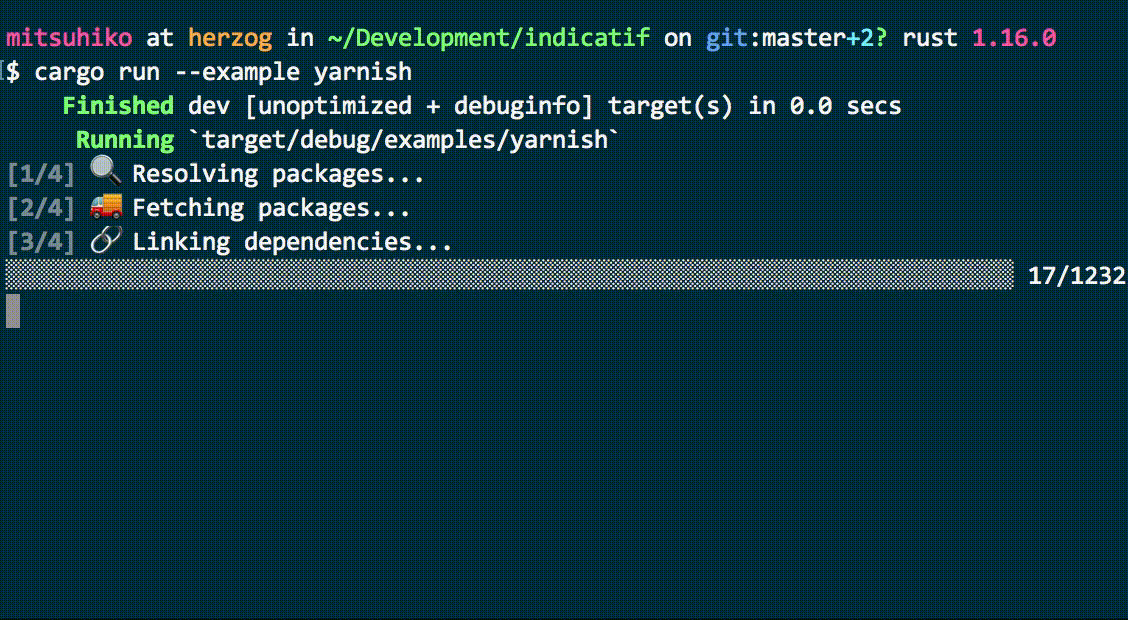
创建一个常规进度条的最小示例代码如下:
use std::thread;
use std::time::Duration;
use indicatif::ProgressBar;
fn main() {
let pb = ProgressBar::new(1024);
for _ in 0..1024 {
pb.inc(1);
thread::sleep(Duration::from_millis(5));
}
pb.finish_with_message("done");
}
首先使用 ProgressBar::new(1024)
实例化进度条,然后使用 inc(1)
方法更新进度条,最后使用 finish_with_message
收尾。
除了常规进度条,还可以创建自旋进度条。在count-files这个项目里,由于需要处理的文件总数是未知的,所以需要使用自旋进度条。
use std::thread;
use std::time::Duration;
use indicatif::ProgressBar;
fn main() {
let pb = ProgressBar::new_spinner();
for _ in 0..1024 {
pb.inc(1);
thread::sleep(Duration::from_millis(5));
}
pb.finish_with_message("done");
}
另外官方文档里对进度条结构的描述如下:
The progress bar is an Arc around its internal state. When the progress bar is cloned it just increments the refcount (so the original and its clone share the same state).
所以,如果你的程序需要在递归函数中更新进度条,可以使用 clone
方法统一操作一个进度条。
最后附上进度条官方代码:
GitHub - console-rs/indicatif at 0.16.x
A command line progress reporting library for Rust - GitHub - console-rs/indicatif at 0.16.x
如果你好奇我如何在递归调用中使用自旋进度条:
GitHub - yinguobing/count-files: A simple command line tool to count all files in a directory.
A simple command line tool to count all files in a directory. - GitHub - yinguobing/count-files: A simple command line tool to count all files in a directory.
Comments ()